I'm playing with iOS using Swift recently. Swift is quite similar to Python that I already know. After reading the syntax briefly, I started writing some code to create classes.
Code
class Vehicle {
var maker: String
init (maker: String) {
self.maker = maker
}
}
class Truck: Vehicle {
var wheels: Int
init (maker: String, wheels: Int) {
super.init(maker: maker)
self.wheels = wheels
}
}
Error
Then I got
Property 'self.wheels' not initialized at super.init call
Solution
This is really confusing, the message makes me think that I should add a wheels
property in the super class (Vehicle
), which doesn't make sense, right? What if I'm adding a Boat
, which doesn't have wheels.
After reading through the documents, the solution turned out to be relatively simple, you make the super.init
after all the initialization of properties in the child class. Therefore instead of above definition, use this:
class Truck: Vehicle {
var wheels: Int
init (maker: String, wheels: Int) {
self.wheels = wheels
super.init(maker: maker) // move after wheels is initialized
}
}
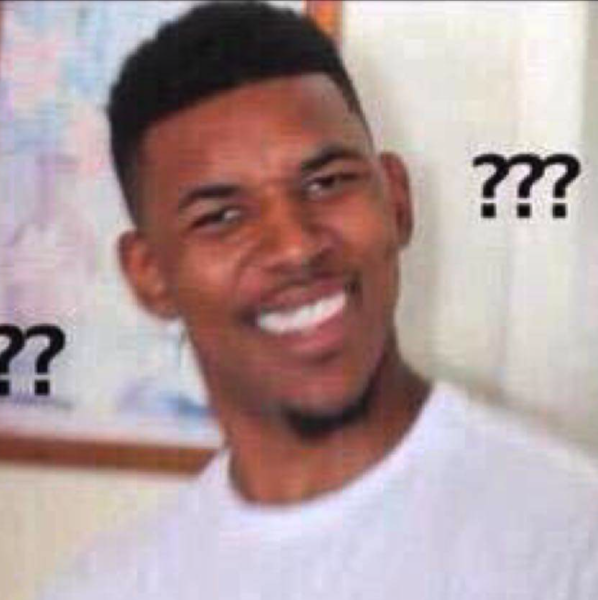